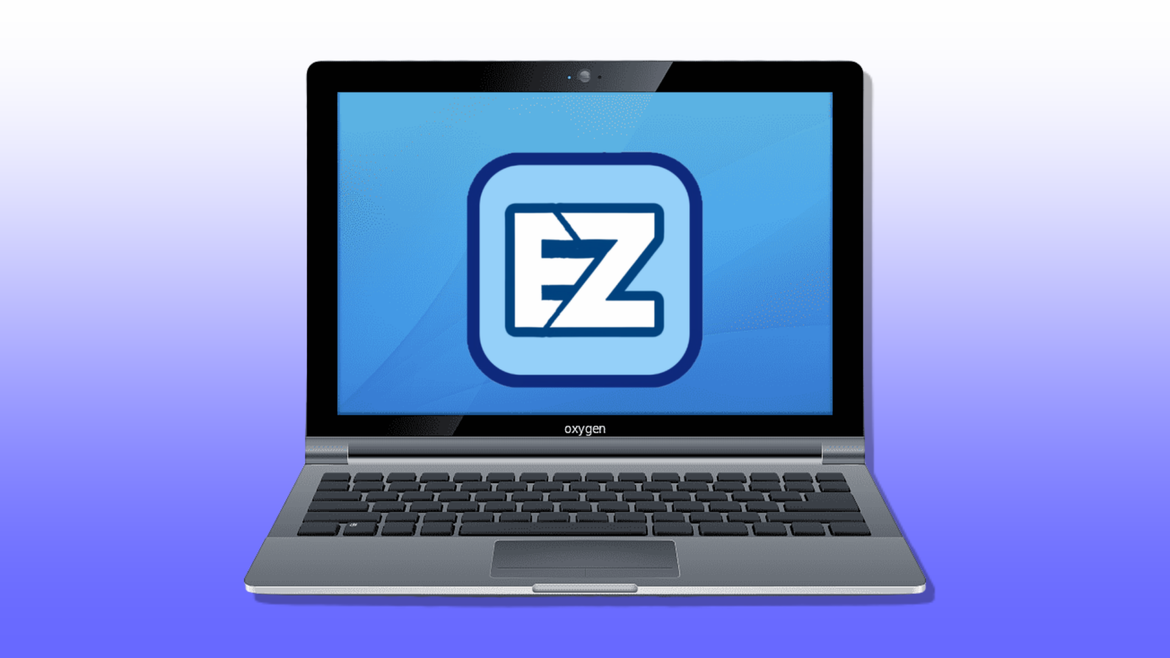
Front-end development is the art of crafting the user interface and experience of a website – everything you see and interact with in your browser. If you're looking to dive into this exciting field and build interactive websites, this guide will walk you through the essential tools and resources.
What is Front-End Development?
Front-end development, also known as client-side development, is the process of creating the visual elements and interactive features of a website or web application that users see and interact with directly. It involves using a combination of programming languages, frameworks, and libraries to build the user interface (UI) and user experience (UX) of a website.
A front-end developer is responsible for implementing the design and functionality of a website, ensuring that it is visually appealing, user-friendly, and responsive across different devices and browsers. They work closely with designers and back-end developers to bring the website to life and create a seamless experience for the end-user.
The Role of a Front-End Developer A front-end developer's responsibilities can vary depending on the specific project and organization, but generally include:
Translating designs into code: Converting visual designs created by UI/UX designers into HTML, CSS, and JavaScript code.
Building user interfaces: Developing interactive and dynamic user interfaces using front-end frameworks and libraries.
Ensuring cross-browser compatibility: Testing and debugging code to ensure websites function consistently across different browsers (Chrome, Firefox, Safari, etc.) and devices (desktops, tablets, smartphones).
- Optimizing website performance: Improving website speed, performance, and scalability.
- Implementing responsive design: Creating websites that adapt to different screen sizes and devices.
- Collaborating with back-end developers: Integrating front-end code with server-side logic and databases.
- Staying up-to-date with new technologies: Keeping abreast of the latest trends, techniques, and best practices in front-end development.
Essential Skills for Front-End Developers
To excel in front-end development, you'll need to acquire a combination of technical and soft skills.
Technical Skills
HTML (Hypertext Markup Language): The foundation of web development, HTML is used to structure the content of a web page. It defines the elements and layout of a website, including headings, paragraphs, images, links, and forms.
CSS (Cascading Style Sheets): CSS is used to control the visual presentation of a website. It defines the styles for HTML elements, such as colors, fonts, layout, and animations. CSS allows developers to create visually appealing and consistent designs across multiple web pages.
JavaScript: A versatile programming language that adds interactivity and dynamic functionality to websites. JavaScript is used to create interactive elements, handle user events, manipulate the DOM (Document Object Model), and communicate with servers.
Front-End Frameworks and Libraries: Frameworks and libraries provide pre-written code and structures to help you build complex web applications more efficiently. Popular choices include React, Angular, and Vue.js.
Responsive Design: The ability to create websites that adapt to different screen sizes and devices is crucial in today's mobile-first world. Front-end developers need to be proficient in using CSS media queries and flexible layouts to ensure websites provide a seamless experience across desktops, tablets, and smartphones.
Version Control (Git): Git is essential for tracking changes to your code, collaborating with others, and managing different versions of your project. Platforms like GitHub, GitLab, and Bitbucket provide hosting for Git repositories and facilitate collaboration.
Browser Developer Tools: Modern browsers come with built-in developer tools that allow you to inspect and debug your code, analyze website performance, and test responsiveness. These tools are invaluable for front-end developers to identify and fix issues, optimize performance, and ensure a smooth user experience.
Web Performance Optimization: Optimizing website speed and performance is crucial for user experience and SEO. Front-end developers should be familiar with techniques such as image optimization, code minification, caching, and lazy loading to ensure websites load quickly and efficiently.
Testing and Debugging: Front-end developers need to be able to test their code thoroughly to identify and fix bugs. This includes unit testing, integration testing, and user acceptance testing. Debugging tools and techniques are essential for troubleshooting issues and ensuring code quality.
Accessibility: Creating websites that are accessible to everyone, including users with disabilities, is an important consideration. Front-end developers should be aware of accessibility guidelines (WCAG) and implement techniques such as semantic HTML, alternative text for images, and keyboard navigation to ensure websites are inclusive.
Soft Skills In addition to technical skills, front-end developers also need strong soft skills to collaborate effectively and succeed in their roles.
Communication: Clear and effective communication is essential for collaborating with designers, back-end developers, and stakeholders. Front-end developers need to be able to explain technical concepts to non-technical people and articulate their ideas and solutions.
Problem-solving: Front-end development often involves solving complex problems and finding creative solutions. Developers need to be able to analyze issues, identify root causes, and develop effective strategies to overcome challenges.
Collaboration: Front-end developers work as part of a team, often with designers, back-end developers, and project managers. The ability to collaborate effectively, share knowledge, and work towards a common goal is crucial.
Time Management: Developers often work on multiple projects with deadlines, so effective time management skills are essential. Prioritizing tasks, managing workload, and meeting deadlines are critical for success.
Attention to Detail: Front-end development requires a keen eye for detail to ensure that websites are visually appealing, pixel-perfect, and function as expected across different browsers and devices.
Continuous Learning: The field of web development is constantly evolving, so front-end developers need to be committed to continuous learning. Staying up-to-date with new technologies, trends, and best practices is essential for staying relevant and competitive.
Essential Tools for Front-End Development
A front-end developer's toolkit includes a variety of software and platforms to write, test, and manage code. Here's a breakdown of the essential tools:
Code Editor: A code editor is where you'll write and edit your HTML, CSS, and JavaScript code. Popular choices include:
Visual Studio Code (VS Code): A free, powerful, and highly customizable editor with a vast library of extensions. VS Code offers features like syntax highlighting, code completion, debugging tools, and integrated Git support.
Sublime Text: A fast and lightweight editor known for its speed and performance. Sublime Text is popular for its clean interface, powerful features, and extensive plugin ecosystem.
Atom: An open-source editor developed by GitHub, offering a good balance of features and customization. Atom is highly customizable and allows developers to tailor the editor to their specific needs.
Version Control System (Git): Git is essential for tracking changes to your code, collaborating with others, and managing different versions of your project.
GitHub: A popular platform for hosting Git repositories and collaborating on projects. GitHub provides a web-based interface for managing Git repositories, collaborating on projects, and contributing to open-source software.
GitLab: Another web-based Git repository manager with features for CI/CD (Continuous Integration/Continuous Deployment). GitLab offers a complete DevOps platform with features for version control, CI/CD, and project management.
Bitbucket: A Git repository management solution, particularly popular for its integration with Atlassian's other tools. Bitbucket is a Git repository management solution that integrates seamlessly with other Atlassian products like Jira and Trello.
Package Managers: Package managers help you manage dependencies (libraries and frameworks) in your projects.
npm (Node Package Manager): The default package manager for Node.js, used for installing and managing JavaScript libraries and frameworks.
Yarn: Another popular package manager for JavaScript, known for its speed and performance.
Task Runners/Build Tools: Task runners and build tools automate repetitive tasks in the development process.
Webpack: A powerful module bundler that bundles JavaScript, CSS, and other assets into optimized files for the browser.
Gulp: A task runner that automates tasks like minification, concatenation, and compilation.
Grunt: Another task runner that automates common development tasks.
Browser Developer Tools: Modern browsers come with built-in developer tools that allow you to inspect and debug your code, analyze website performance, and test responsiveness. These are crucial for any front-end developer. You can usually access them by pressing F12, or right-clicking on a page and selecting "Inspect".
Testing Frameworks: Testing frameworks help you write and run tests to ensure your code is working correctly.
Jest: A popular JavaScript testing framework developed by Facebook, commonly used for testing React applications.
Mocha: A flexible JavaScript testing framework that can be used with various assertion libraries.
Cypress: An end-to-end testing framework for testing anything that runs in a browser.
Front-End Frameworks and Libraries
Frameworks and libraries provide pre-written code and structures to help you build complex web applications more efficiently. Here are some of the most popular choices:
React: A JavaScript library for building user interfaces, particularly single-page applications. React is known for its component-based architecture, virtual DOM for performance, and large community. React allows developers to break down complex UIs into smaller, reusable components, making it easier to manage and update code.
Angular: A comprehensive framework developed by Google for building complex web applications. Angular provides a robust set of features, including routing, dependency injection, and a powerful templating system. Angular is a full-fledged framework that provides a complete solution for building large-scale applications.
Vue.js: A progressive framework for building user interfaces. Vue.js is known for its simplicity, ease of learning, and flexibility. It can be used for both small projects and large-scale applications. Vue.js is a lightweight and versatile framework that can be easily integrated into existing projects.
Choosing the Right Framework/Library
The choice of framework or library depends on several factors, including the project's complexity, team size, and specific requirements.
React: Ideal for building dynamic and interactive single-page applications (SPAs). React's component-based architecture and large community make it a popular choice for many projects.
Angular: A robust framework suitable for large-scale enterprise applications. Angular provides a complete solution with a steep learning curve but offers a structured approach to development.
Vue.js: A versatile and approachable framework that can be used for both small and large projects. Vue.js is a good choice for developers who want a balance of simplicity and flexibility.
Learning Resources
To master these tools and frameworks, consider the following online learning resources:
Codecademy: Offers interactive courses and career paths in front-end development, covering HTML, CSS, JavaScript, React, and more. Codecademy provides a structured learning path with hands-on exercises and projects.
freeCodeCamp: A non-profit organization that provides free coding courses, certifications, and a supportive community. freeCodeCamp offers a comprehensive curriculum, including HTML, CSS, JavaScript, and various front-end frameworks.
MDN Web Docs (Mozilla Developer Network): A comprehensive resource for web developers, with documentation, tutorials, and examples for HTML, CSS, JavaScript, and web APIs. MDN is an invaluable resource for web developers of all levels, providing accurate and up-to-date information.
YouTube: A vast resource for video tutorials on front-end development. Search for specific topics, frameworks, or tools to find relevant content. Many developers and educators share their knowledge on YouTube, offering a wide range of tutorials and courses.
Udemy/Coursera: These platforms offer a wide range of paid courses on front-end development, often taught by industry experts. Udemy and Coursera provide structured courses with video lectures, assignments, and certificates of completion.
Additional Tips for Aspiring Front-End Developers
Build Projects: The best way to learn front-end development is to build projects. Start with simple projects and gradually increase the complexity as you improve your skills.
Contribute to Open Source: Contributing to open-source projects is a great way to gain experience, collaborate with other developers, and build your portfolio.
Build a Portfolio: Create a portfolio website to showcase your projects and skills to potential employers.
Network with Other Developers: Attend meetups, conferences, and online communities to connect with other developers, learn from their experiences, and find job opportunities.
Stay Updated: The field of web development is constantly evolving, so it's important to stay updated with the latest trends, technologies, and best practices.
Conclusion Front-end development is a dynamic and rewarding field that offers numerous opportunities for creative and technical individuals. By mastering the essential tools, technologies, and skills, you can build a successful career as a front-end developer and create engaging and user-friendly web experiences.
Add comment
Comments